LG202: IoT Cloud Server Project - Class Library
Now we have our database securely set-up we need to be able to access it. I reminisced in my introduction, about the good old days when Dreamweaver wrote inline code for me, I have also discussed some of the security issues around allowing systems direct access to data.
We have tied our data down and the only way to interact with it is through CRUD Stored Procedures.
We will be using that data in many places throughout the system, by creating a Class Library to handle that interaction, we can create a level of abstraction that ensures we are never in a position where we find ourselves running a Find & Replace on a system that currently has 20,000 lines of code! One point of failure, one place to correct code.
With the widespread use of the cloud and microservices, architecture has become modular. By separating the functionality into a class library, we could create a new library that used a NoSQL database instead of SQL, without having to change any code in the WebApp or any other applications that are using it, simply by replacing the Library reference in the project.
To get a list of all the users in any application we simply use List
Accidental Damage
As the use of IoT and AI becomes more prevalent in our society, data integrity will be vital. Using a strongly typed programming language such as C# and Class Libraries for data manipulation offers a high level of protection.
Talking about the importance of Class Libraries in Taligent Technology’s system architecture (link) back in 1995, Tim Cotter commented that, “Encapsulation enforces data abstraction: the organization of data into small, independent objects that can communicate with each other. Encapsulation protects the data in an object from accidental damage, but allows other objects to interact with that data by calling the object's member functions.” This is as true now, as it was then, we can distribute the WezSocket library to other developers, knowing if they use it we have data integrity.
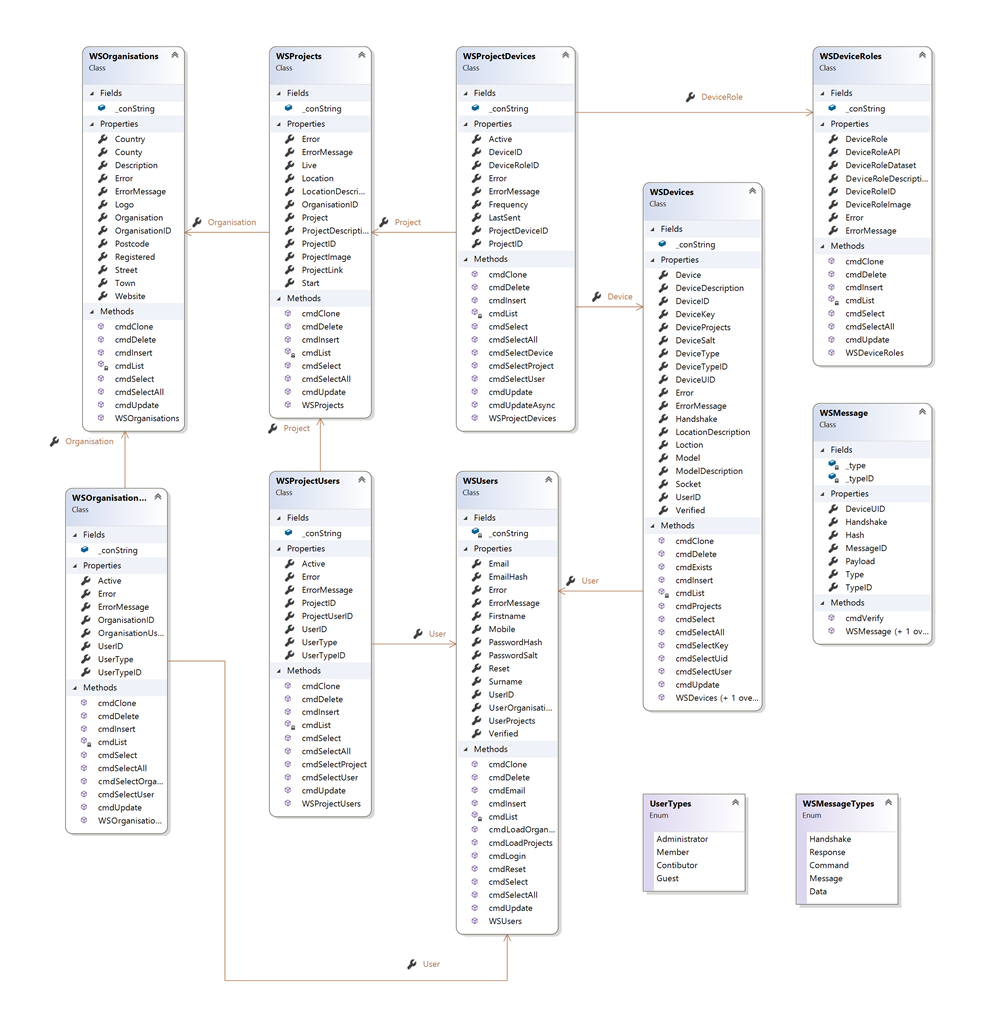
The Anatomy of a WezSocket Class
The Classes generated by the Stored Procedure in the Database, are simple and effective. The easiest way to explain them is to share the annotated code which I have done below.
Where we need to pull related records through, or include hashing or encryption there is still some work to be done, but again, by encapsulating all the encryption methods into another class we achieve a level of abstraction, where the commands are used. We could swap an entire encryption algorithm in one place to update the entire system.
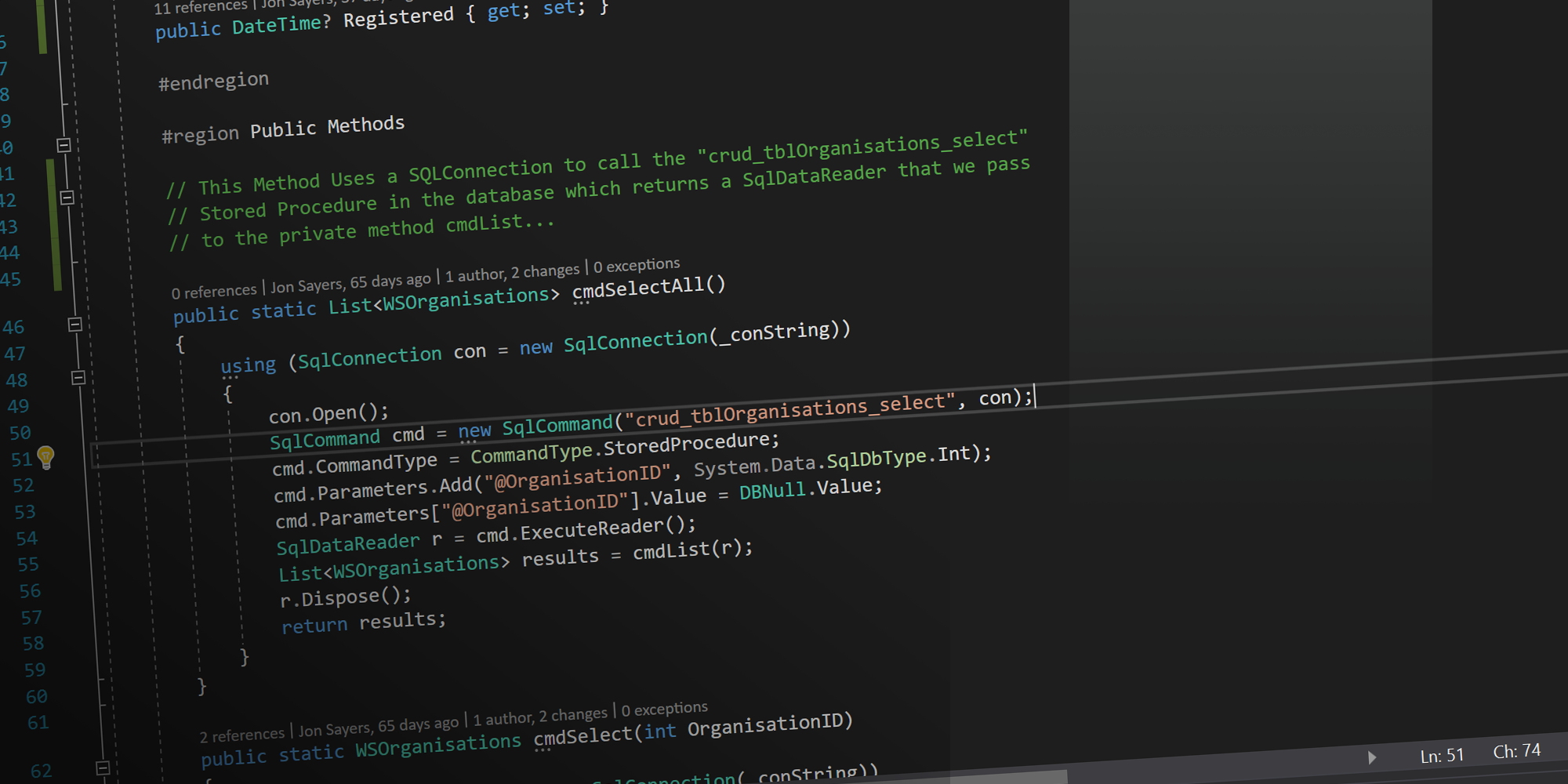
The SelectAll method in the class file (top) passes a null value to the Stored Procedure (bottom).
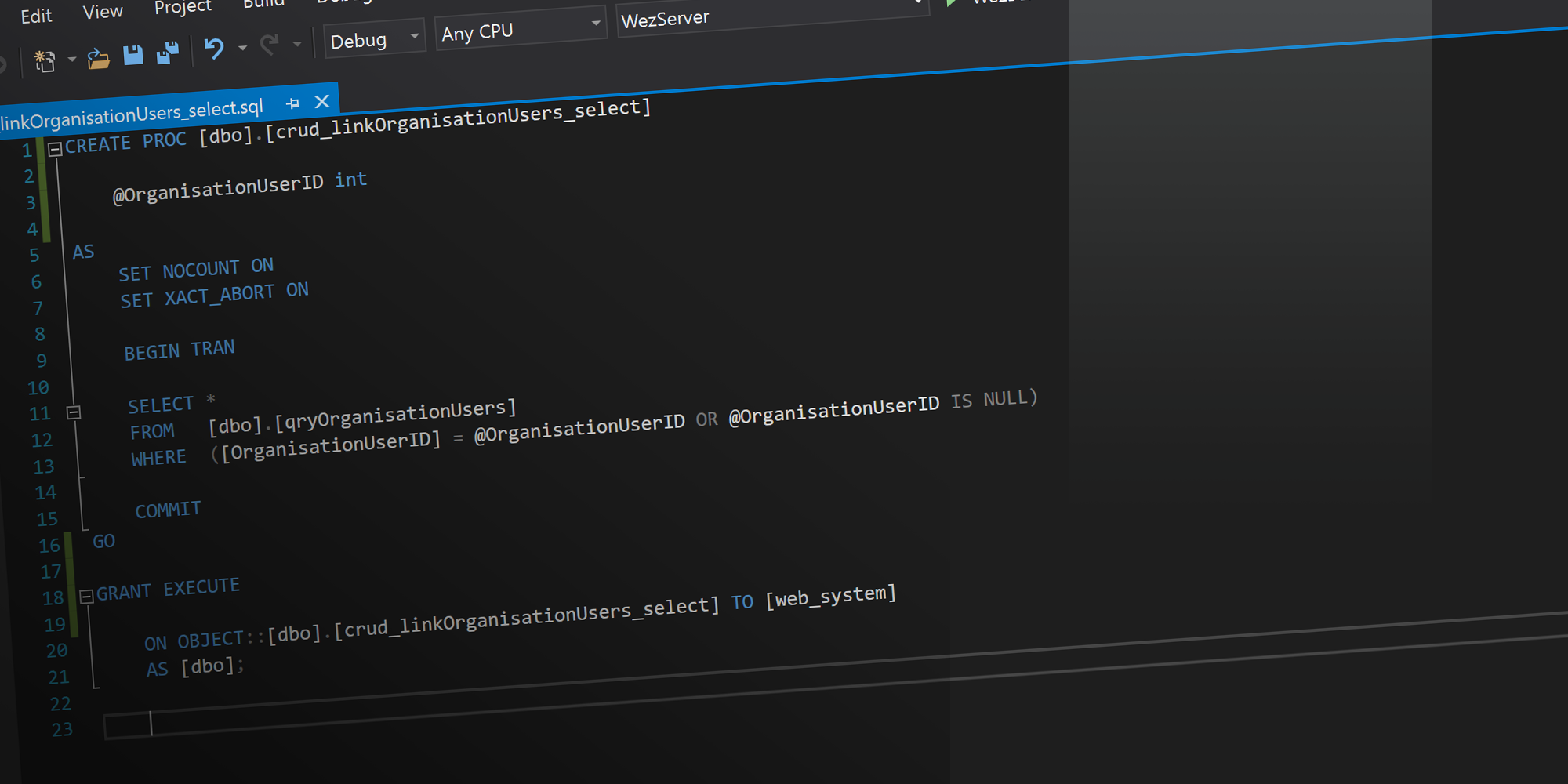
Data Hashing & Encryption
As I mentioned in the last article, we will be hashing information we only need to prove and encrypting sensitive information. We will be performing these functions in the Class Library and sending the hashed/encrypted data to the database.
Here comes a bold statement… “Any website that allows you to retrieve your password, is not secure!” I don’t care how many pets’ names, maiden names or favourite teachers they ask you, and if they send it to you by email, post or pigeon. If they can retrieve it for you, that means it can be retrieved by someone, which means they are encrypting it, not hashing it.
They don’t need to know your password, I’ll explain why…
Let’s say your password is number one on the most used passwords list, ‘123456’. When you register with my website and save your password, I’m going to use a one-way hashing algorithm on it called MD5, your password isn’t ‘123456’ it’s ‘e10adc3949ba59abbe56e057f20f883e’. You entered ‘123456’ in the web form but it saved the hashed value, you’ll have to enter ‘123456’ to log-in and the system will hash this and check it matches the hashed value in the database. I will never know what you entered in the web form.
Except, I will, won't I… you used the most commonly used password in the world… maybe I have a table with the 10,000 most used passwords in that I’ve also MD5 hashed… how long do you think it will take me to find your password.
The only attack against a hashed password is a brute force attack, as a user on this informative Stack Exchange thread states, “…if I have H and I want to find P such that Hash(P) = H, then the only way is to find P is to make a guess, compute the hash of this guess, and see if it's equal to H.” But to find the most common password in the world, we’re not going to need to be very brutal! https://security.stackexchange.com/questions/38166/methods-used-to-reverse-a-hash
So what do we, well, we save a unique string, or Salt, to another field in the database that would have been a really good password, for example, ‘E22BD30F51E8BA1B67705DF339C3796CB7’ and we add that to your password before we hash it. Now the record of your password is unique, so the hacker can’t compare the 100 most popular hashes in our database with his Hash Dictionary each individual record now has to be brute-forced individually and even though the Salt is available to the hacker, he doesn’t know how we have combined it with the password, and what other elements we’ve added to the string.
Always salt your hash!
We can’t just hash all sensitive data, as we need to use some of it, for example, we need to be able to email users. To encrypt our data we will use the Rijndael symmetric-key-algorithm, know as AES, or Advanced Encryption Standard. With a 256bit key, the sun should swallow the earth before our data is vulnerable, I’m going to write much more about this in the future, but for now, we have done a lot to ensure things are secure, as long as we look after our Keys!
So now I’ll sleep at night, we can get on with the job in hand, creating a WebSocket Server.
There's a link to my encryption class and extensions below.